- 浏览: 637081 次
-
文章分类
最新评论
-
涛声依旧是:
怎么提示掉线,怎么才能在线
基于Tomcat7、Java、WebSocket的服务器推送聊天室 -
dcode:
楼主写的不错,正好遇到点问题,看着你的文章解决了,感谢分享。。 ...
在 Visual Studio 2010 中配置SharpPcap
iphone-实现页面的切换和修改
//
// movie.h
// day04
//
//
// Copyright (c) 2012年 __MyCompanyName__. All rights reserved.
//
#import <Foundation/Foundation.h>
@interface movie : NSObject <NSCopying, NSCoding>
{
NSString* name;
int price;
NSString* summary;
}
@property(nonatomic,copy) NSString* name;
@property(nonatomic,assign)int price;
@property(nonatomic,copy)NSString* summary;
-(id)init;
-(id)initWithName:(NSString*)_name andPrice:(int)_price andSummary:(NSString*)_summary;
-(void)encodeWithCoder:(NSCoder *)aCoder;
-(id)initWithCoder:(NSCoder *)aDecoder;
-(NSComparisonResult)compareName:(id)element;
-(NSComparisonResult)comparePrice:(id)element;
-(NSComparisonResult)compareSummary:(id)element;
@end
//
// movie.m
// day04
//
//
// Copyright (c) 2012年 __MyCompanyName__. All rights reserved.
//
#import "movie.h"
@implementation movie
@synthesize name;
@synthesize price;
@synthesize summary;
-(id)init{
self=[super init];
if(!self){
return nil;
}
name=[[NSString alloc] initWithFormat:@"zhang"];
if(!name){
[self release];
return nil;
}
// [name autorelease];
price=15;
summary=[[NSString alloc] initWithFormat:@"asdf"];
if(!summary){
[self release];
return nil;
}
// [summary autorelease];
return self;
}
-(id)initWithName:(NSString*)_name andPrice:(int)_price andSummary:(NSString*)_summary{
if(!_name||_price<0||!_summary){
NSLog(@"--------------");
[self release];
return nil;
}
self=[super init];//这个地方应该是调用谁的初始化方法
if(!self){
return nil;
}
name=[[NSString alloc] initWithFormat:@"%@",_name];
if(!name){
[self release];
return nil;
}
//[name autorelease];
price=_price;
summary=[[NSString alloc] initWithFormat:@"%@",_summary];
if(!summary){
[self release];
return nil;
}
//[summary autorelease];
return self;
}
-(NSString*)description{
NSString* ns= [[NSString alloc]initWithFormat:@"name:%@,price:%d,summary:%@",name,price,summary];
[ns autorelease];
return ns;
}
-(void)dealloc{
[name release];
[summary release];
[super dealloc];
}
-(id)copyWithZone:(NSZone *)zone{
movie* m=[[movie allocWithZone:zone]initWithName:@"adsfadf" andPrice:1 andSummary:@"qer"];
return m;
}
-(void)encodeWithCoder:(NSCoder *)aCoder{
[aCoder encodeObject:name forKey:@"name"];
[aCoder encodeInt:price forKey:@"price"];
[aCoder encodeObject:summary forKey:@"summary"];
}
-(id)initWithCoder:(NSCoder *)aDecoder{
if((self=[super init])){
self.name=[aDecoder decodeObjectForKey:@"name"];
self.price=[aDecoder decodeIntForKey:@"price"];
self.summary=[aDecoder decodeObjectForKey:@"summary"];
}
return self;
}
-(NSComparisonResult)compareName:(id)element
{
//NSString* str1 = [NSString stringWithString:name];
//NSString* str2 = [NSString stringWithString:[element name]];
//return [str1 compare:str2];
return [name compare:[element name]];
}
-(NSComparisonResult)comparePrice:(id)element{
NSNumber* n1=[NSNumber numberWithInt:price];
NSNumber* n2=[NSNumber numberWithInt:[element price]];
return [n1 compare:n2];
}
-(NSComparisonResult)compareSummary:(id)element{
return [summary compare:[element summary]];
}
@end
//
// AppDelegate.h
// Iphome_day01
//
//
// Copyright (c) 2012年 __MyCompanyName__. All rights reserved.
//
#import <UIKit/UIKit.h>
@class ViewController;
@interface AppDelegate : UIResponder <UIApplicationDelegate>
@property (strong,nonatomic)UINavigationController* navagate;
@property (strong, nonatomic) UIWindow *window;
@property (strong, nonatomic) ViewController *viewController;
@end
//
// AppDelegate.m
// Iphome_day01
//
//
// Copyright (c) 2012年 __MyCompanyName__. All rights reserved.
//
#import "AppDelegate.h"
#import "ViewController.h"
@implementation AppDelegate
@synthesize window = _window;
@synthesize viewController = _viewController;
@synthesize navagate=_navagate;
- (void)dealloc
{
[_window release];
[_viewController release];
[super dealloc];
}
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
self.window = [[[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]] autorelease];
// Override point for customization after application launch.
self.viewController = [[[ViewController alloc] initWithNibName:@"ViewController" bundle:nil] autorelease];
_navagate = [[UINavigationController alloc]initWithRootViewController:self.viewController];
[self.window addSubview:_navagate.view];
self.window.rootViewController = self.viewController;
[self.window makeKeyAndVisible];
return YES;
}
- (void)applicationWillResignActive:(UIApplication *)application
{
/*
Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
Use this method to pause ongoing tasks, disable timers, and throttle down OpenGL ES frame rates. Games should use this method to pause the game.
*/
}
- (void)applicationDidEnterBackground:(UIApplication *)application
{
/*
Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
*/
}
- (void)applicationWillEnterForeground:(UIApplication *)application
{
/*
Called as part of the transition from the background to the inactive state; here you can undo many of the changes made on entering the background.
*/
}
- (void)applicationDidBecomeActive:(UIApplication *)application
{
/*
Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
*/
}
- (void)applicationWillTerminate:(UIApplication *)application
{
/*
Called when the application is about to terminate.
Save data if appropriate.
See also applicationDidEnterBackground:.
*/
}
@end
//
// ViewController.h
// Iphome_day01
//
//
// Copyright (c) 2012年 __MyCompanyName__. All rights reserved.
//
#import <UIKit/UIKit.h>
@class movie;
@interface ViewController : UIViewController
{
movie * mo;
UILabel* label1;
UILabel* label2;
UILabel* label3;
}
@property(nonatomic,retain)movie *mo;
@property(nonatomic,retain) IBOutlet UILabel* label1;
@property(nonatomic,retain) IBOutlet UILabel* label2;
@property(nonatomic,retain) IBOutlet UILabel* label3;
-(IBAction)Edit:(id)sender;//实现
//系统会每次都调用该方法
- (void)viewWillAppear:(BOOL)animated;
@end
//
// ViewController.m
// Iphome_day01
//
//
// Copyright (c) 2012年 __MyCompanyName__. All rights reserved.
//
#import "ViewController.h"
#import "movie.h"
#import "EditViewController.h"
@implementation ViewController
@synthesize mo;
@synthesize label1;
@synthesize label2;
@synthesize label3;
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Release any cached data, images, etc that aren't in use.
}
#pragma mark - View lifecycle
- (void)viewDidLoad
{
mo=[[movie alloc]initWithName:@"龙门飞甲" andPrice:200 andSummary:@"古装片"];
self.title=@"电影详情";
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)viewDidUnload
{
label1=nil;
label2=nil;
label3=nil;
mo=nil;
[super viewDidUnload];
// Release any retained subviews of the main view.
// e.g. self.myOutlet = nil;
}
- (void)viewWillAppear:(BOOL)animated
{
label1.text= mo.name;
label2.text=[NSString stringWithFormat:@"%d",mo.price];
label3.text=mo.summary;
[super viewWillAppear:animated];
}
- (void)viewDidAppear:(BOOL)animated
{
[super viewDidAppear:animated];
}
- (void)viewWillDisappear:(BOOL)animated
{
[super viewWillDisappear:animated];
}
- (void)viewDidDisappear:(BOOL)animated
{
[super viewDidDisappear:animated];
}
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
// Return YES for supported orientations
return (interfaceOrientation != UIInterfaceOrientationPortraitUpsideDown);
}
-(void)dealloc{
label1=nil;
label2=nil;
label3=nil;
[mo release];
[super dealloc];
}
-(IBAction)Edit:(id)sender{
EditViewController *edit=[[EditViewController alloc]initWithNibName:@"EditViewController" bundle:nil];
edit.editMovie=mo;
/*
UIModalTransitionStyleCoverVertical = 0,
UIModalTransitionStyleFlipHorizontal,//翻转
UIModalTransitionStyleCrossDissolve,//渐变
#if __IPHONE_OS_VERSION_MAX_ALLOWED >= __IPHONE_3_2
UIModalTransitionStylePartialCurl,//翻页
*/
edit.modalTransitionStyle=UIModalTransitionStyleFlipHorizontal;
// [self presentModalViewController:edit animated:YES ];//UIViewController 的压栈方法
[self.navigationController pushViewController:edit animated:YES];//导航UINavigationController的压栈方法
[edit autorelease];
NSLog(@"edit called!!!");
}
@end
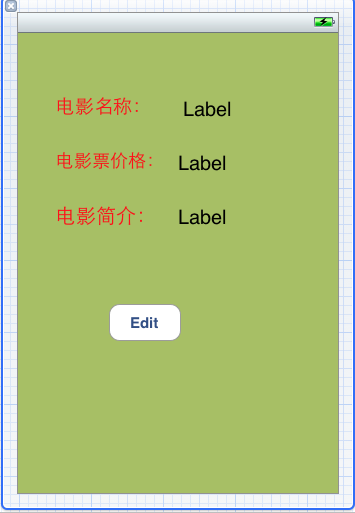
//
// EditViewController.h
// Iphome_day01
//
//
// Copyright (c) 2012年 __MyCompanyName__. All rights reserved.
//
#import <UIKit/UIKit.h>
#import "movie.h"
@class ViewController;
@interface EditViewController : UIViewController <UITextFieldDelegate>
{
movie* editMovie;
UITextField *NameField;
UITextField *PriceField;
UITextField *SummaryField;
}
@property(nonatomic,retain) IBOutlet UITextField* NameField;
@property(nonatomic,retain) IBOutlet UITextField* PriceField;
@property(nonatomic,retain) IBOutlet UITextField* SummaryField;
@property(nonatomic,retain)movie* editMovie;
-(BOOL)textFieldShouldReturn:(UITextField *)textField;//去除键盘
-(IBAction)Back:(id)sender;
@end
//
// EditViewController.m
// Iphome_day01
//
//
// Copyright (c) 2012年 __MyCompanyName__. All rights reserved.
//
#import "EditViewController.h"
#import "ViewController.h"
#import "movie.h"
@implementation EditViewController
@synthesize NameField;
@synthesize PriceField;
@synthesize SummaryField;
@synthesize editMovie;
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)didReceiveMemoryWarning
{
// Releases the view if it doesn't have a superview.
[super didReceiveMemoryWarning];
// Release any cached data, images, etc that aren't in use.
}
#pragma mark - View lifecycle
- (void)viewDidLoad
{
NSLog(@"%@",editMovie);
NameField.text=editMovie.name;
PriceField.text=[NSString stringWithFormat:@"%d", editMovie.price];
SummaryField.text=editMovie.summary;
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
}
- (void)viewDidUnload
{
NameField=nil;
PriceField=nil;
SummaryField=nil;
[super viewDidUnload];
// Release any retained subviews of the main view.
// e.g. self.myOutlet = nil;
}
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
// Return YES for supported orientations
return (interfaceOrientation == UIInterfaceOrientationPortrait);
}
-(IBAction)Back:(id)sender{
// [self dismissModalViewControllerAnimated:YES];
[self.navigationController popToRootViewControllerAnimated:YES];
NSLog(@"back called!!");
}
-(void)dealloc{
[NameField release];
[PriceField release];
[SummaryField release];
[super dealloc];
}
//去掉键盘
-(BOOL)textFieldShouldReturn:(UITextField *)textField{
[textField resignFirstResponder];
return YES;
}
-(void)textFieldDidEndEditing:(UITextField *)textField{
if(NameField==textField){
//把textfield的文本给name赋值
editMovie.name=textField.text;
}
else if(PriceField==textField){
editMovie.price=[textField.text intValue];
}else{
editMovie.summary=textField.text;
}
NSLog(@"%@",editMovie);
}
@end
相关推荐
在使用一些移动端的App或PC端的软件的时候,我们常常会有一些界面之间切换的操作,尤其是在移动端的设备上,因为屏幕尺寸和交互方式的特性,就更多的会出现这些切换的操作,而很突然地从一个界面切换到另一个界面会...
注意:导航到另一个页面、更改选项卡或使用任何应用程序切换器(或 Alt-Tab)切换到另一个应用程序同样会退出全屏模式。 了解更多 从 <= 2.3.5 迁移 成分 一般情况下,可以简单地使用双向绑定切换全屏状态,...
默认的移动视图是带有iOS 11的iPhone,但您可以从选项页面进行更改。只需添加所需的用户代理即可将移动视图更改为所需的视图。有许多网站提供各种设备的用户代理字符串。只需将所需的副本复制并粘贴到选项页面的指定...
稍后会更新2.x版本的使用小技巧安卓版的识兔,密码123更新日志识别图片发布图片瀑布流保存图片跨页面修改首页图片点赞功能webView的加载样式适应iPhoneX和安卓异形屏登录切换路由/登录重定向页面各种细节处理设计...
PP浏览器覆盖了iOS移动操作系统,适用于iPhone/iPad/iTouch手机,是首款提供下载音视频功能,首创播放器离线观看,集下载与解压常用几十种压缩包直接预览,超级解压,使浏览器不仅仅是浏览器更成为下载资源管理必备...
新增 查看主题的时候,可以选择“精简”模式,并可自由切换。精简模式下,内容为王 新增 发起投票的时候,可以同时上传图片,从而针对图片进行投票 新增 版块列表、看帖页面的用户名的颜色,根据用户组设定,进行...
iPhone中的页面间切换动画效果比较漂亮,今天学习了下其中一个类似翻页的动画效果,并经过修改做成类似地图卷起一角的那种形式。效果如下:
1.3.4 “认我测”质检服务平台的设计和实现 8 1.4 本文的结构安排 8 第二章 多窗口类浏览器设计 11 2.1 多窗口类浏览器需求分析 11 2.1.1 Activity简介 11 2.1.2 Fragment简介 11 2.1.3 多窗口类浏览器需求 12 2.2 ...
新增iPhone和Android手机客户端下载地址可通过扫描二维码实现下载(更加方便的实现下载到手机了) 新增后台可设置附件类型 修复网站安全问题(感谢 秒杀@360 、 合肥滨湖虎子@360 的反馈) 修复发布某些字符的...
• 添加、编辑和管理幻灯片切换 幻灯片中的高级功能 • 另存为 .ppt • 创建并编辑动画 • 向幻灯片添加注释 • 拍照并插入相机照片 • 设置文档密码 OfficeSuite PDF – PDF 编辑器 • 打开 PDF 文件 • 管理多个...
首页有个 评论/最新文章/热门文章 切换卡的标题是英文的(有的人以为是没汉化),这个标题其实也是在小工具里,自己输入的,所以你要自己去小工具输入修改标题。FlyingNews 新闻杂志 wordpress主题 2014.10.31 更新...
新增iPhone和Android手机客户端下载地址可通过扫描二维码实现下载(更加方便的实现下载到手机了) 新增后台可设置附件类型 修复网站安全问题(感谢 秒杀@360 、 合肥滨湖虎子@360 的反馈) 修复发布某些字符的...
默认的移动视图是带有iOS 13的iPhone,但您可以从选项页面进行更改。 只需添加所需的用户代理即可将移动视图更改为所需的视图。 有许多网站提供各种设备的用户代理字符串。 只需将所需的副本复制并粘贴到选项页面的...
修复:Iphone手机浏览上下滑动页面会产生停滞效果 修复:手机端地图定位自动跳转至后台设定坐标 修复:手机端同步 会员过期后 上架职位提示升级会员 引导消费 修复:手机端企业注册无法自动分配企业顾问 修复:...
Top -page.ru这是一项服务,允许您保存和同步书签,无论您如何欣赏设备和浏览器:带Windows,iOS(iPhone,iPad,iPod touch)或Mac OS的笔记本电脑或个人计算机。现在您可以在设备之间切换,而不是混淆任何内容。...
自识别浏览器语言,智能切换对应语言 自建栏目 网站栏目随意创建、删除、修改 网站以后升级灵活方便,不拘一格 内置功能模块 页面、产品、新闻、评论、招聘等 每个功能模块都可随意扩展字段 自定义表单 无...
Top-Page.ru是一项服务,允许您保存和同步书签,无论使用哪种设备和浏览器:使用Windows,iOS(iPhone,iPad,iPod touch)或Mac OS的笔记本电脑或个人电脑。现在你可以在不同的设备之间切换 如果您突然决定在其中一...
如今,市场上已经有了许多移动平台,包括Symbian、iPhone、Windows Mobile、BlackBerry、Java Mobile Edition和Linux Mobile(LiMo)等。当我向别人说起Android时,他们的第一个疑问通常是:我们为什么还需要另一...
首页有个 评论/最新文章/热门文章 切换卡的标题是英文的(有的人以为是没汉化),这个标题其实也是在小工具里,自己输入的,所以你要自己去小工具输入修改标题。 FlyingNews 新闻杂志 wordpress主题 2014.9.26 更新...